How to use Prism EventAggregator for .NET app development
In the second part of this developer series, we explain how to use Prism EventAggregator in .NET app development and Xamarin apps.
5 min. read - April 6, 2020
In my previous article, I covered why to use Prism EventAggregator for .NET app development, the Publisher-Subscriber pattern, and the scenarios in which to use it. Now it’s time to show exactly how to use it with some code!
Installation?
Well, there isn’t any! The EventAggregator is part of Prism.Core, so if you’re already using Prism in your project, the EventAggregator is ready and waiting for you.
Inject it into the constructor
EventAggregator implements the interface IEventAggregator
. To use it in any class (that’s registered with Prism’s IoC), all you need to do is ask for an instance of IEventAggregator
in the constructor.
1
2
3
4
Prism will automatically pass an instance of its EventAggregator when the ViewModel is constructed. There’s no need to register or set up anything else.
Create an event
Next, we need something to send: an event. There are two types of events: events WITHOUT a parameter and events WITH a parameter. To create an event without a parameter, we create a regular new class and have it inherit from PubSubEvent
.
1
2
3
If we want to pass a parameter, we inherit from the generic PubSubEvent<T>
where T
is the type of the parameter we want to pass. This can be a primitive type or a complex object.
1
2
3
Subscribe to an event
Now that we have our EventAggregator and our event, it’s time to subscribe to it. First, we need to call GetEvent<TEvent>()
on our EventAggregator, which will return an instance of our event. Then we call Subscribe(…)
on the event passing in the method or Action that will handle our event.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
It’s similar for handling events with parameter:
1
2
3
4
5
6
7
8
9
10
11
12
13
Publish an event
Now that our events will be handled, all that’s left is to publish them. To do this, we again have to call GetEvent<TEvent>()
to create an instance of the event we want to publish. This time we’ll call Publish(…)
.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
Or, pass a parameter:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
Unsubscribe from an event
Finally, if you want to stop listening to an event, you can unsubscribe from it easily with the Unsubscribe()
method:
1
2
3
Advanced stuff
Everything described until now should be enough to cover 95% of cases. However, if you want some more control, EventAggregator has you covered. Namely, the Subscribe() method has a few optional parameters that we can use to configure EventAggregator’s behavior:
threadOption
: An enum that specifies on which thread the event will be handled. It has three values: PublisherThread (default), UIThread and BackgroundThread.keepSubscriberReferenceAlive
: A bool that’s false by default. Set this to true if you want the EventAggregator to keep a reference of a subscriber, even if it has no other references, which prevents it from being garbage collected. (This will make it behave in a similar way as Xamarin.MessagingCenter does.)filter
: A filter in the form of a lambda expression returning a bool that evaluates whether or not the subscriber should receive the event. (This is only applicable for events that have a parameter.)
Here you can see them all in action:
1
2
3
4
5
6
7
Next up: EventAggregator unit tests
As you can see, EventAggregator is a powerful tool that’s easy to use. If you want a complete working example, check out the repository here.
In the next article, I’ll cover writing unit tests that include the EventAggregator. Make sure to follow us on Facebook, Twitter, and LinkedIn to be notified about the next post!
Article Author:
Subscribe for more insights
Get our newsletter in your inbox.
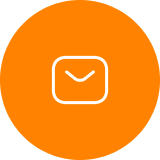
Contact us.
Let's build something lovable. Together.
We help companies of all sizes build lovable apps, websites, and connected experiences.