Python test automation framework for apps and websites
ArcTouch’s developers built a versatile and open-source test automation framework in Python for apps and websites. Free download.
5 min. read - November 18, 2020
As a QA Engineer, I am a huge fan of test automation for apps and websites. The hardest part of deploying test automation is building something from scratch that can meet the specific needs of each project. Recently, we built a test automation framework in Python that is flexible enough to use for most web and app projects — and simple enough even if you’re not an expert in coding.
The ArcTouch QA team is using our Python test automation framework on several projects now — from our clients’ iOS and Android apps to the web, and even on our own website. What used to take us about five hours to create for each project, now takes less than an hour with our test automation framework.
And we’re happy to share it! See the link to the GitHub repo at the bottom of this post.
Why test automation matters
QA engineers spend a lot of time running thousands of regression tests on each new code release — regardless of how big the update. Regression testing is tedious work and error prone, as testers may miss a tiny detail that can cause big problems. Test automation is a development team’s best friend. It’s a series of automated tests that check the expected behavior of the features to the actual outcomes. If the outcomes are aligned, the likelihood of any bugs is greatly reduced.
Why we chose Python for our test automation framework
Flexibility was a huge driver for this project. We needed a test automation framework that allowed us to follow best practices across our diverse digital projects.
Using Python for the test automation framework gives us this versatility. And Python has the added benefit of it being a fairly easy programming language, making it much simpler for developers to customize the framework to meet the needs of each project.
Our test automation framework key features
We include these key features in our Python test automation framework:
Integration with Cucumber: Run behavior-driven development tests with Cucumber.
Parallel testing: Run multiple tests in parallel.
Cross-browser testing: Run tests across Chrome, Safari, Firefox, and Microsoft Edge.
Cross-device testing: Run tests on computers and mobile devices using the same code.
Cross-platform testing: Run tests across native mobile apps and web applications.
Open source: Fully developed with free and open-source tools and assets.
Mock server: Use an integrated mock server for the test executions.
Low maintenance: Minimal time needed to build and maintain tests.
Writing a test in our test automation framework
So, what does an actual test look like in our test automation framework? The structure divides the functionalities and their respective Gherkin scenarios. Cucumber provides the ability to have two business layers, using Gherkin (plain language) and Step Definitions. Each scenario’s step has its equivalence inside the step file, as shown below. Besides using best practices, this structure provides clear organization and readability.
1
2
3
4
5
1
2
3
4
5
Using a page object model
In the code we have access to the current context that provides the location information, the web driver, and other aids for the execution of the tests. The steps access the pages of each feature.
By using a page object model, we add organization and distinction to our tests. The pages inherit general methods of search and interaction previously implemented. The pages contain information about each element being automated as well as their locators that may vary between platforms, like this:
1
2
3
4
5
6
The navigation flow is a page’s role. It contains information from its previous and following pages (see below). All the navigation logic is located at the base page, which helps minimize the amount of maintenance.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
How we use our Python test automation framework
Our Python test automation has already been used in several projects at ArcTouch, including our own website. With each new feature for the site — and with every content update — our site automatically runs a series of regression tests on the staging environment. When these tests pass, our production site is updated. When they don’t, we can easily identify the root cause of the failure.
We have an integration with Slack that notifies everyone in our site-development channel about the status of updates and test results:
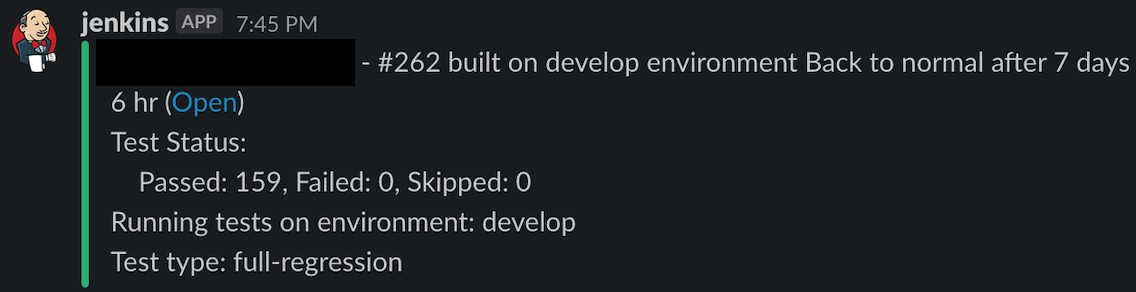
Want to try it out? Download our Python test automation framework
We’re excited to share the ArcTouch Python automation framework in our GitHub repo. Follow the instructions for installing and using the framework in the README.md of the repository.
The framework is configured to run test scenarios using the public sample applications in the project. When installing all the dependencies, you should be able to configure the devices available on your machine through our devices list generator.
Note: For now, this framework is for developers using macOS, as we do here at ArcTouch.
By all means, let us know what you think. Also, if you’re a developer interested in working with ArcTouch, please check out our latest open positions on our careers page. And if you are a business that needs help building great applications, contact us to set up a free consultation.
Article Author:
Subscribe for more insights
Get our newsletter in your inbox.
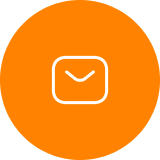
Contact us.
Let's build something lovable. Together.
We help companies of all sizes build lovable apps, websites, and connected experiences.