Why you should use Prism EventAggregator in .NET app development
Our Xamarin app development team explains when and why C# developers should use Prism EventAggregator for .NET app development.
5 min. read - April 3, 2020
Prism is an extensive library for app development in .NET that includes many great features to make your life as a C# developer so much easier. One of these features is the EventAggregator
. In this post, I’ll explain what the EventAggregator
is, why it’s so useful, and where you should use it.
What is Prism EventAggregator?
In short, EventAggregator
is Prism’s implementation of the Publisher-Subscriber pattern. Publisher-Subscriber is a messaging pattern designed to facilitate asynchronous communication in your application. Specifically, it resolves the common problem of sending messages between components that are difficult, impractical, or impossible to link.
At its core, the pattern consists of events that are published to an event bus, which in turn passes them to one or more subscribers. The subscribers can handle the event in any way they see fit.
You can find more detailed information here.
A simple representation of the Publisher-Subscriber pattern
Why use Prism EventAggregator for .NET app development?
The Publisher-Subscriber model has various benefits:
Decoupled: Publishers and Subscribers don’t know about each other.
Asynchronous: The Publisher can quickly send a message and continue with its own work, without having to wait for the Subscriber to finish handling the event.
Separation of concerns: Publishers don’t need to know what Subscribers do with the event, and Subscribers don’t need to know where an event comes from.
Scalable: No matter how many publishers, subscribers, and events you have, you only ever need a reference to one event bus.
EventAggregator
has all these benefits and adds a few more that are especially helpful in .NET app development:
Prevent memory leaks:
EventAggregator
will NOT keep a reference of any subscribers that were disposed. There’s no reason to unsubscribe manually.Send complex objects: Send complex objects as a parameter when publishing an event to give the subscriber more context and info when handling the event.
Thread flexibility: Choose which thread to handle incoming events.
Filter events: Subscribers can choose which events to process and which to ignore.
When to use Prism EventAggregator?
To recap, Publisher-Subscriber is a messaging pattern designed for communication between components that are difficult or impractical to link. In a Prism project, EventAggregator
is often used to send messages between two or more ViewModels, or between services that don’t have a reference to each other. Another use case is when one event needs to be handled by many different subscribers, and it wouldn’t be practical to pass a publisher reference to each of these subscribers.
EventAggregator in Xamarin.Forms
If you’re familiar with Xamarin.Forms, you may be thinking, “Wait, doesn’t Xamarin.Forms already have something like this?” And you’d be right — Xamarin.Forms has its own implementation of Publisher-Subscriber, namely MessagingCenter. But I prefer Prism’s EventAggregator
for these reasons:
Memory leaks: MessagingCenter will keep a reference to any disposed subscribers if you don’t unsubscribe manually. (Just Google “Xamarin MessagingCenter memory leaks” and you’ll find many forum posts about performance issues.)
Flexibility: MessagingCenter doesn’t have thread flexibility or event filters like
EventAggregator
does.Testability: MessagingCenter uses static methods, which makes writing unit tests more difficult. Prism, on the other hand, will automatically inject an
EventAggregator
instance into the constructor, so you can mock it easily.
A few words of warning
I hope by now you’re convinced of the flexibility and utility of EventAggregator
, but as always, there are some things to watch out for.
Although EventAggregator
holds only weak references to subscribers, it’s still possible that it will hold a strong reference IF the publisher passes a parameter AND the subscriber holds a reference to that parameter. So if you need to do this, it’s a good idea to unsubscribe manually when your subscriber is disposed.
Also, while the decoupled structure of EventAggregator
is great, it can lead to increased complexity when overused. There may be a separation of concerns between publisher and subscriber, but there isn’t between the events themselves (they’re all kept in the same EventAggregator
). In large projects with many events, this may obscure communication flows. My advice is to only use EventAggregator
where it’s necessary. Don’t structure your whole project around it. Like with everything in life, moderation is key.
Follow us to learn more about Prism EventAggregator
There are many reasons to use EventAggregator
in your Prism project and for .NET app development. In an upcoming post, we’ll explain HOW to use it with code samples. So make sure to follow us on Facebook, Twitter, and LinkedIn to be notified about it.
Article Author:
Subscribe for more insights
Get our newsletter in your inbox.
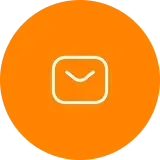
Contact us.
Let's build something lovable. Together.
We help companies of all sizes build lovable apps, websites, and connected experiences.